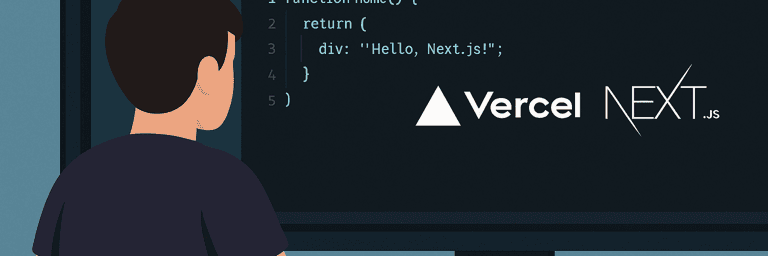
Getting Started with Next.js: From Setup to Deployment
Next.js has become one of the most popular React frameworks for building modern web applications. In this guide, I'll walk you through creating a new Next.js project, running it locally, and deploying it to Vercel.
What is Next.js?
Next.js is a React framework that provides features like server-side rendering, static site generation, API routes, and more out of the box. It's designed to make building React applications easier and more productive with a great developer experience.
Prerequisites
Before we start, make sure you have the following installed:
- Node.js (version 18.17 or later)
- npm, yarn, or pnpm (I'll use npm in this tutorial)
- A code editor (VS Code, WebStorm, etc.)
- Git (for deployment)
Creating a New Next.js Project
Let's start by creating a new Next.js project. Open your terminal and run:
npx create-next-app@latest my-next-app
This will prompt you with a few questions about your project setup:
✓ Would you like to use TypeScript? … Yes
✓ Would you like to use ESLint? … Yes
✓ Would you like to use Tailwind CSS? … Yes
✓ Would you like to use [src](http://_vscodecontentref_/0) directory? … Yes
✓ Would you like to use App Router? … Yes
✓ Would you like to customize the default import alias? … No
Answer according to your preferences. I recommend using TypeScript and the App Router for new projects.
Project Structure
After the installation completes, navigate to your project directory:
cd my-next-app
Let's look at the key files and directories in our project:
app/
: Contains the application routes and components (with App Router)public/
: Static assets like images and fontsnext.config.js
: Configuration file for Next.jspackage.json
: Project dependencies and scriptstsconfig.json
: TypeScript configuration (if you chose TypeScript)
Running the Application Locally
To start the development server, run:
npm run dev
This will start your application at http://localhost:3000. Open this URL in your browser to see your application.
Making Changes
Let's modify the home page. Open app/page.tsx
(or page.js
if not using TypeScript) and change some content.
For example, replace the existing content with:
export default function Home() {
return (
<main className="flex min-h-screen flex-col items-center justify-between p-24">
<div className="z-10 max-w-5xl w-full items-center justify-between font-mono text-sm">
<h1 className="text-4xl font-bold mb-6">Welcome to My Next.js App</h1>
<p className="text-xl">This is my first Next.js application!</p>
</div>
</main>
);
}
Save the file, and the browser should automatically update with your changes.
Building for Production
When you're ready to deploy your application, you need to build it first. Run:
npm run build
This command creates an optimized production build of your application. Next.js will show you a summary of the build, including information about the generated pages, routes, and bundle sizes.
To test the production build locally, run:
npm run start
This will start a production server at http://localhost:3000.
Deploying to Vercel
Vercel, created by the same team behind Next.js, is the easiest way to deploy Next.js applications.
Setting Up Vercel
- If you don't have a Vercel account, go to vercel.com and sign up.
- Install the Vercel CLI:
npm install -g vercel
- Login to Vercel from the CLI:
vercel login
Follow the prompts to complete the login process.
Deploying Your Application
There are two main ways to deploy to Vercel:
Option 1: Using Vercel CLI
From your project directory, run:
vercel
This will guide you through the deployment process with a series of prompts. For most cases, you can accept the default settings.
Option 2: Connecting to a Git Repository
For a more streamlined workflow:
- Push your project to a Git repository (GitHub, GitLab, or Bitbucket).
- Login to your Vercel dashboard at vercel.com.
- Click "New Project" and import your repository.
- Configure your project settings and click "Deploy".
Once deployed, Vercel will provide you with a URL where you can access your application. The platform automatically creates deployments for each push to your repository, enabling continuous deployment.
Conclusion
Congratulations! You've successfully created, built, and deployed a Next.js application. Next.js offers many more features to explore, such as API routes, server-side rendering options, image optimization, and more.
As you continue your Next.js journey, be sure to check out the official documentation at nextjs.org/docs for more detailed information and advanced features.
Happy coding!